Web methods
Most methods of ReglaWebService return a value of type MethodResult.
Upon successful login the Login(...) method returns a token (through Methodresult.Messages[0]). This token is used as a first parameter to all other methods.
The element in MethodResult.Messages[] with the key INFO_RUNNING_TIME contains the number of milliseconds it took to run the method.
Following is a description of each method and code examples grouped by usage categories.
Error checking in code examples is kept to a minimum.
Data shown in screenshots may be different from the current state of database.
The screenshots themsleves are not necessarily up to date.
Some of the examples use this method (and constant) to display messages:
Some of the examples use this method to display value from MethodResult.Messages[] by key:
All the code examples have the same structures.
The actual code example is located at //Code example goes here....
Various Methods
Method | Parameters | Description |
DownloadFile | String token Enum.FileType fileType int referenceID out MethodResult result |
Download a file.This can either be a project accounting entry signature, an accounting transaction attachment or a company system or form logo. The logos do not require a reference id. This method returns an object of type File. |
GetCompany | String token out MethodResult result |
Get the company of a logged in user. This method returns an object of type Company. |
GetCountries | String token out MethodResult result |
Get all countries. This method returns an array of strings. |
GetCultures | String token String country out MethodResult result |
Gets all available cultures for a particular country. This method returns an array of strings. |
GetCurrencies | String token out MethodResult result |
Get all currencies. This method returns an array of objects of type Currency. |
GetCurrency | String token String currencyCode out MethodResult result |
Get a currency object by currency code. This method returns an object of type Currency. |
GetCurrencyByDate | String token String currencyCode Datetime date out MethodResult result |
Get a currency object by currency code and date. This method returns an object of type Currency. |
GetCurrentDateTime | String token out MethodResult result |
Returns current date and time from server. |
GetDefaultPaymentMethod | String token out MethodResult result |
Get the default payment method as set in sale controls. This method returns an object of type PaymentMethod. |
GetDimension | String token Int dimensionId out MethodResult result |
Get a dimension object by id. This method returns an object of type Dimension. If a dimension is not found an empty dimension (one with all values zeros and empty strings) is returned. |
GetDimensions | String token int groupId out MethodResult result |
Get all dimensions by group id. This method returns an array of objects of type Dimension. |
GetEmployee | String token String employeeNumber out MethodResult result |
Get employee by employee number. This method returns an object of type Employee. |
GetEmployees | String token out MethodResult result |
Get all employees. This method returns an array of objects of type Employee. |
GetLanguage | String token int languageID out MethodResult result |
Get a language object by language id. This method returns an object of type Language. |
GetLanguages | String token out MethodResult result |
Get all languages. This method returns an array of objects of type Language. |
GetPaymentMethod | String token int paymentMethodID out MethodResult result |
Get a payment method object by payment method id. The language of the result depends on the language of logged in user. This method returns an object of type PaymentMethod. |
GetPaymentMethods | String token out MethodResult result |
Get all payment methods. The language of the result depends on the language of logged in user. This method returns an array of objects of type PaymentMethod. |
GetPostalCode |
String token String postalCodeValue out MethodResult result |
Get a postal code object by postal code value. This method returns an object of type PostalCode. |
GetPostalCodes | String token out MethodResult result |
Get all postal codes. This method returns an array of objects of type PostalCode. |
GetProperty | String token Property property out MethodResult result |
Get various values assignable via control pages in Regla. |
GetUser | String token out MethodResult result |
Get the logged in user. This method returns an object of type User. |
GetVatDefinition | String token String vatDefinitionKey out MethodResult result |
Get a vat definition object by vat definition key. This method returns an object of type VatDefinition. |
GetVatDefinitions | String token out MethodResult result |
Get all vat definitions. This method returns an array of objects of type VatDefinition. |
Login | String username String password |
Upon successful login MethodResult.Messages[0] contains a token needed for all other method calls. This method returns an object of type MethodResult. |
LoginWithComment | String username String password String comment |
The comment is written to Regla‘s log. |
UploadFile | String token File file |
Upload a file. This method returns an object of type MethodResult. |
ValidateFile | String token File file |
Validate a file. This method returns an object of type MethodResult. |
ValidateToken | String token | This method returns a bool value indicating whether token is valid or not. |
AuthenticatePassword | String token Property property String password out MethodResult result |
Authenticates password for certain actions. The following action is supported: SalePasswordForCashRegisterReport. Returns true if parameter password is correct. The setting for the password is done in www.regla.is. |
Get Payment Methods
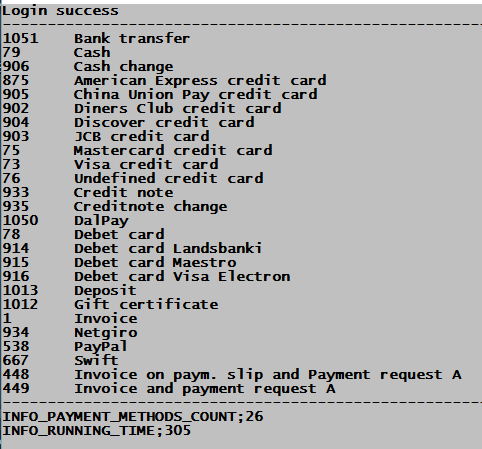
Get Payment Method

Customer
Method | Parameters | Description |
GetCustomer | String token String customerNumber out MethodResult result |
Get a customer object by customer number. This method returns an object of type Customer. |
GetCustomerExtendedAttributes | String token String customerNumber out Boolean deliveryNoteAsDefault out Boolean showPriceOnDeliveryNote |
Gets Boolean valuesstating whether customer gets delivery note as default type of invoice and whether price will be shown on delivery a delivery note. Both values are returned as out parameters. |
GetCustomerGroup | String token String groupNumber Boolean includeCustomers out MethodResult result |
Gets a customer group by group number. Customers that belong to the group can be included. This method returns an object of type CustomerGroup. |
GetCustomerGroups | String token Boolean includeCustomers out MethodResult result |
Gets all customer groups. Customers that belong to the group can be included. This method returns an array of objects of type CustomerGroup. |
GetDefaultCustomer | String token out MethodResult result |
Get the default customer as set in sale controls. This method returns an object of type Customer. |
SaveCustomer | String token Customer customer |
This method inserts a customer if it does not already exist. If customer already exists it is updated if needed. The CreateInvoice(…) and CreateSubscriptionEntry(…) methods both call this method internally. This method returns an object of type MethodResult |
SearchCustomers | String token String search int indexFrom int maxRecordCount out MethodResult result |
Searches active company customers by search string. Multiple search values can be separated by ",", ";" or "+". An empty search string returns all customers. The search string can contain parts of customer names and customer numbers. indexFrom is a zero based start index. maxRecordCount defines how many records to return max. The result array contains the current page number (INFO_SEARCH_PAGE_NUMBER), total page count (INFO_SEARCH_PAGE_COUNT), the total number of customers (INFO_SEARCH_TOTAL_COUNT) and number of customers returned by this call to the method (INFO_SEARCH_RECORD_COUNT), respectively. This method returns an array of objects of type Customer. |
SearchCustomersModified | String token String search DateTime modified int indexFrom int maxRecordCount out MethodResult result |
This method adds a from time to method SearchCustomers(…) and only returns customers that have changed since that time. This method returns an array of objects of type Customer. |
ValidateCustomer | String token Customer customer |
The CreateInvoice(…) and CreateSubscriptionEntry(…) methods both call this method internally. This method returns an object of type MethodResult. |
Get Customer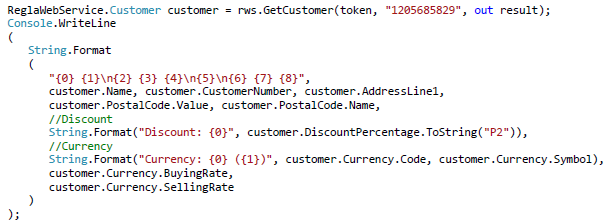
Save customer

Validate Customer
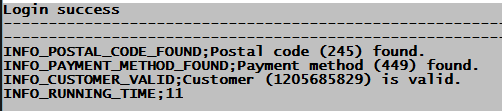
Search customer
The company in the following code example has a total of eigth customers.
They are listed three on each page, resulting in three pages.
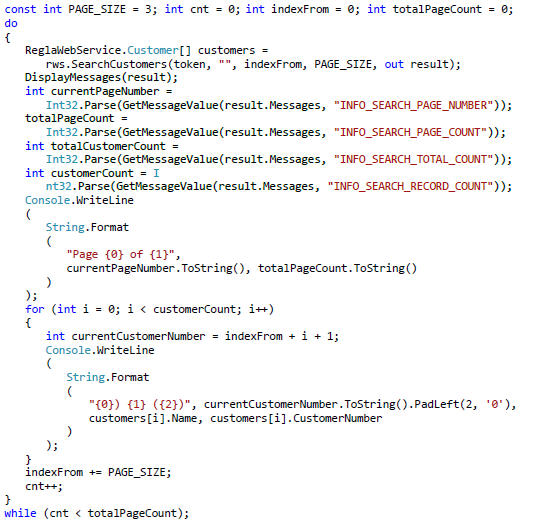
Product
Method | Parameters | Description |
AddBarcodeToProduct | String token String barcode String productNumber |
Adds a barcode to a product. |
DownloadProductImages | String token String productNumber out MethodResult result |
Download all images for a particular product. This method returns an array of objects of type File. |
DownloadProductImagesWithSize | String token String productNumber int width int height out MethodResult result |
Download images for a particular product, resized to a specific width and height. This method returns an array of objects of type File. |
GetCombinations | String token String productNumber out MethodResult result |
Gets the next level product combinations for a given product number (parent product). This method returns an array of objects of type Combination. |
GetProduct | String token String productNumber out MethodResult result |
Get a product object by product number. This method returns an object of type Product. |
GetProductByBarcode | String token String barcode out MethodResult result |
Get a product object by barcode. This method returns an object of type Product. |
GetProductDiscount | String token String productNumber String customerNumber DateTime when out String description out Decimal discountPercentUsed out Boolean customerDiscountTooHigh out MethodResult result |
Get the discount for a product. Customer discount can exceed product max discount. In that case product max discount is used. This method returns a decimal value. |
GetProductDiscountAndPriceNow | String token String productNumber String customerNumber out Decimal productPrice out Decimal discountPercentUsed out Boolean customerDiscountTooHigh out String priceDescription out String discountDescription out MethodResult result |
Get both product price and discount as it is at this moment. This method returns a decimal value, the product discount. The product price is returned as an out variable. |
GetProductDiscountAndPriceWhen | String token String productNumber String customerNumber DateTime when out Decimal productPrice out Decimal discountPercentUsed out Boolean customerDiscountTooHigh out String priceDescription out String discountDescription out MethodResult result |
The same as method GetProductDiscountAndPriceNow(…) except that the caller provides the reference time in parameter when. |
GetProductGroup | String token String groupNumber Boolean includeProducts out MethodResult result |
Gets product group by group number. Parameter includeProducts determines whether to get products associated with group. This method returns an array of objects of type ProductGroup. |
GetProductGroups | String token Boolean includeProducts out MethodResult result |
Gets all product groups. Parameter includeProducts determines whether to get products associated with group. This method returns an object of type ProductGroup. |
GetProductPrice | String token String productNumber String customerNumber DateTime when out String description out MethodResult result |
Get product price taking into account things lke customer discount, product rate etc. This method return a decimal number. |
GetProductRates | String token out MethodResult result |
Get all product rates. This method returns an array of objects of type ProductRate. |
GetProductRatesOrOfferValidInUse | String token out MethodResult result |
Returns true if company is using product rates or has a valid offer. |
RemoveBarcodeFromProduct | String token string barcode String productNumber |
Removes a barcode from a product. |
SaveProduct | String token Product product |
This method inserts a product if it does not already exist. If product already exists it is updated if needed. The CreateInvoice(…) and CreateSubscriptionEntry(…) methods both call this method internally. This method returns an object of type MethodResult. |
SearchProducts | String token String search int indexFrom int maxRecordCount out MethodResult result |
Searches active company products by search string. Multiple search values can be separated by ",", ";" or "+". An empty search string returns all products. The search string can contain parts of product names, product numbers, barcodes, product group numbers and product group names. indexFrom is a zero based start index. maxRecordCount defines how many records to return max. The result array contains the current page number (INFO_SEARCH_PAGE_NUMBER), total page count (INFO_SEARCH_PAGE_COUNT), the total number of products (INFO_SEARCH_TOTAL_COUNT) and number of products returned by this call to the method (INFO_SEARCH_RECORD_COUNT), respectively. This method returns an array of objects of type Product. |
SearchProductsModified | String token String search DateTime from int indexFrom int maxRecordCount out MethodResult result[] |
This method adds a from time to method SearchProducts(…) and only returns products that have changed since that time. It is also considered a change if barcode, product group or vat definition for the product has changed. This method returns an array of objects of type Product. |
UpdateBarcodeForProduct | String token String barcode String productNumber String newBarcode |
Updates a barcode for a product. |
ValidateProduct | String token Product product |
The CreateInvoice(…) and CreateSubscriptionEntry(…) methods both call this method internally. This method returns an object of type Product. |
Get Product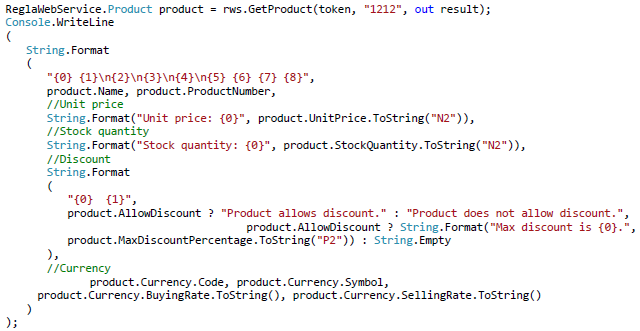
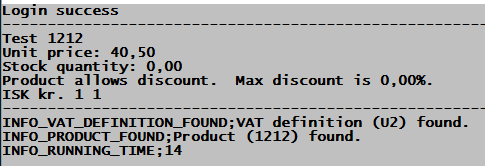
Invoice and InvoiceEntry
Method | Parameters | Description |
CancelBankClaim | String token int invoiceNumber String bankUser String bankPassword |
Cancels a bank claim by invoice number. Protection of bank user name and password are the responsibility of the consuming company. |
CreateCreditInvoice | String token Invoice originalInvoice DateTime creditInvoiceDate String creditInvoiceComment |
Credits an invoice. Credit invoice date cannot be in the future, not before the date of its original invoice and not in a period closed in Regla. |
CreateCreditInvoiceWithPayments | String token Invoice originalInvoice DateTime creditInvoiceDate String creditInvoiceComment PaymentPartition[] creditPayments |
Credits an invoice. Payment partitions for the credit invoice can be different from the original invoice. |
CreateCreditInvoiceWithPaymentsAndFiscalData | String token Invoice originalInvoice DateTime creditInvoiceDate String creditInvoiceComment PaymentPartition[] creditPayments String fiscalTerminalId String fiscalControlCode int fiscaltReceiptId |
Credits an invoice. Payment partitions for the credit invoice can be different from the original invoice. |
CreateInvoice | String token Invoice invoice |
As well as creating the invoice an accounting booking is made, invoice is sent by email if the customer has an invoice email registered and a bank claim is created if the customer payment method requires it. The customer, as well as all invoice entries (along with their products), are validated and saved (product unit price is not saved) before creating the invoice itself. Price calculation is done with four decimals. An invoice must contain at least one invoice entry. This method returns an object of type MethodResult. |
CreateInvoiceWithUpdateAndValidationOptions | String token Invoice invoice Boolean updateCustomer Boolean updateProducts Boolean validateInvoice |
This method adds the options whether or not to update customer, update products and validate invoice, to method CreateInvoice(…). |
DeleteSavedInvoice | String token String invoiceNumber |
Deletes a saved invoice. |
GetInvoice | String token String invoiceNumber out MethodResult result |
Get an invoice object by invoice number. This method returns an object of type Invoice. |
GetInvoiceByType | String token String invoiceNumber Enum.InvoiceType invoiceType out MethodResult result |
Get an invoice by type. |
GetInvoicePDF | String token String invoiceNumber out MethodResult result |
Gets an invoice as a pdf-file. This method returns a byte array (byte[]). |
GetInvoiceURL | String token String invoiceNumber out MethodResult result |
Gets an url for an invoice. This method returns a string. |
GetLocationGroups | String token bool includeLocations out MethodResult result |
Gets all location groups. This method returns an array of object of type LocationGroup. |
GetPosReport | String token DateTime from DateTime to Int linewidth String posID out MethodResult result |
Get POS report for a particular period. This method returns an xml formatted string. |
GetPosReportByDepartmentID | String token DateTime from DateTime to Int linewidth String posID int departmentID out MethodResult result |
Get POS report for a particular period and department. This method returns an xml formatted string. |
GetPosReportByDepartmentIDPasswordProtected | String token DateTime from DateTime to Int linewidth String posID int departmentID String password out MethodResult result |
Get POS report for a particular period and department. Password is required. This method returns an xml formatted string. |
GetPosReportPasswordProtected | String token DateTime from DateTime to Int linewidth String posID String password out MethodResult result |
Get POS report for a particular period. Password is required. This method returns an xml formatted string. |
GetSavedInvoice | String token String invoiceNumber MethodResult result |
Get a saved invoice. |
GetTaxFree | String token String invoiceNumber int lineWidth out int taxFreeAmount out String taxFreeReferenceCode out Boolean runningOutOfReferenceCodes out MethodResult result |
Get a tax free report for an invoice. This method returns a xml formatted string that represents a TaxFree slip. |
NetgiroConfirmPayment | String token String transactionId Boolean authenticated String customerAuthenticationToken out MethodResult result |
Confirm a Netgiro payment. |
NetgiroCreatePayment | String token Decimal amount int paymentOptionId int paymentCount String customerId Invoice invoice out MethodResult result |
Create a Netgiro payment. |
NetgiroGetPaymentOptions | String token out MethodResult result |
Get Netgiro payment options. |
NetgiroGetPaymentPlans | String token Decimal amount out MethodResult result |
Get Netgiro payment plans. |
SaveInvoice | String token Invoice invoice |
Save an invoice. |
SaveInvoiceWithUpdateAndValidationOptions | String token Invoice invoice Boolean updateCustomer Boolean updateProducts Boolean validateInvoice |
This method adds the options whether or not to update customer, update products and validate invoice, to method SaveInvoice(…). |
SearchInvoices | String token String search int indexFrom int maxRecordCount out MethodResult result |
Searches invoices for a company. Multiple search values can be separated by ",", ";" or "+". An empty search string returns all invoices. The search string can contain parts of invoice numbers, customer names, customer numbers and concering texts. indexFrom is a zero based start index. maxRecordCount defines how many records to return max. The result array contains the current page number (INFO_SEARCH_PAGE_NUMBER), total page count (INFO_SEARCH_PAGE_COUNT), the total number of invoices (INFO_SEARCH_TOTAL_COUNT) and number of invoices returned by this call to the method (INFO_SEARCH_RECORD_COUNT), respectively. This method returns an array of objects of type Invoice. |
SearchInvoicesByType | String token String search Enum.InvoiceType invoiceType int indexFrom int maxRecordCount out MethodResult result |
This method adds invoice type to method SearchInvoices(…). |
SearchInvoicesByTypeWithIncludeOptions | String token String search Enum.InvoiceType invoiceType int indexFrom int maxRecordCount Boolean includeInvoiceEntries out MethodResult result |
This method adds the option whether to include invoice entries to method SearchInvoiceByType(…) |
SearchSavedInvoices | String token String search int indexFrom int maxRecordCount out MethodResult result |
Search saved invoices. |
SearchSavedInvoicesByType | String token String search Enum.InvoiceType invoiceType int indexFrom int maxRecordCount out MethodResult result |
This method adds invoice type to method SearchSavedInvoices(…). |
SearchSavedInvoicesByTypeWithIncludeOptions | String token String search Enum.InvoiceType invoiceType int indexFrom int maxRecordCount Boolean includeInvoiceEntries out MethodResult result |
This method adds the option whether to include invoice entries to method SearchSavedInvoiceByType(…) |
SendBankClaim | String token int invoiceNumber String bankUser String bankPassword |
Sends a bank claim for an invoice to the bank. Protection of bank user name and password are the responsibility of the consuming company. The following rules apply when sending a bank claim: - Bank login must be valid. - Company must have at least one claim info type defined. - Invoice must be of type invoice (not offer, preregistration, delivery note etc.). - Invoice must have a total amount above zero. - Invoice can‘t be a credit invoice. - Invoice can‘t have been paid already. - Invoice can‘t have been sent as a bank claim to the bank already. If a bank claim has not been created already it is created regardless of the rules. The bank claim is not sent if any of the above rules are broken. This method returns an object of type MethodResult. |
SendInvoiceByEmail | String token String invoiceNumber String emailAddress |
Send an invoice via email. Multiple recipients are separated by semicolon. |
UpdateStatusForInvoiceEntry | String token String GUID Enum.InvoiceEntryStatus invoiceEntryStatus DateTime date |
Update invoice entry status, This method returns an object of type MethodResult. |
Create Invoice
The following code examples demonstrate various ways to create an Invoice.
Change Customer Payment Method
Create Invoice, change Customer Payment Method.
Change Product Unit Price
Create Invoice, change Product Unit Price.
This requires the product to have Allow Price Overwrite checked.
Method CreateInvoice(...) calls method SaveProduct(...) but does not change the unit price.
Check Product Stock Quantity
Check if Product is in stock before creating an Invoice.
Customer Discount and Product Max Discount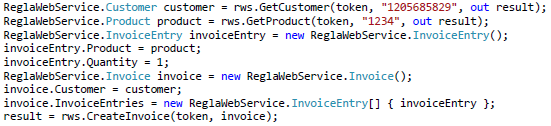
Customer Discount Percentage is 10%.
Customer Discount Percentage is 10% but product max discount is 5%.
Product Max Discount Percentage is used since customer discount exceeds it.
Invoice Entry Discount
Set discount for each Invoice Entry, either as a Percentage or as an Amount, respectively
Currency
Unit price overwrite when customer currency and product currency differ
The following rules apply when registering an invoice via Regla:
- Customer currency becomes the currency for the whole invoice.
- Different currency can be selected from user interface.
- A product that has a different currency is calculated to customer currency.
- Overwritten unit price is considered to be in customer currency,
The webservice acts differently for the last item in the list.
The webservice uses the product currency for the overwritten unit price.
This means that a client is calling the webservice must use: ...but not:
...when unit price is overwritten.
Additional Attachments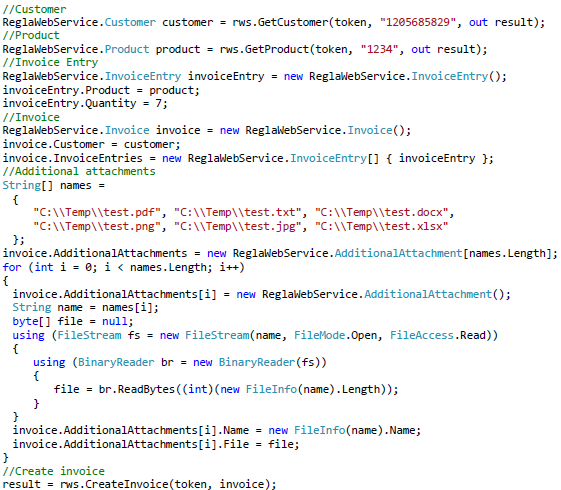
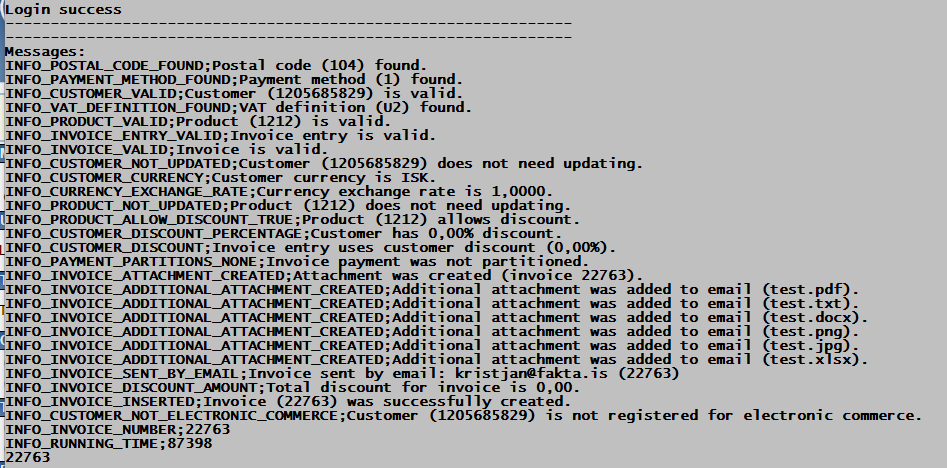
Partitioned payments
The payment for the following invoice is split into four partitions.
40% of the total invoice amount is paid by cash (ISK), 15% by a Mastercard creditcard (EUR) and 45% by a Visa creditcard (EUR).
The Company Exchange Rate Markup is used to calculate the exchange rate for the Euros.
This calculation is only used as a reference, it is up the company consuming ReglaWebService to determine how currency exchange rates are calculated.
The same applies to matching the invoice total amount to the total amount of all payment partitions.
Subscription and SubscriptionEntry
Method | Parameters | Description |
CreateSubscriptionEntry | String token SubscriptionEntry subscriptionEntry |
Depreciated, user SaveSubscriptionEntry(…) instead: Customer, Product and Recipient are all validated and saved before creating the Subscription Entry. A Subscription object is created if it does not already exist, base on Title. This method returns an object of type MethodResult. |
DoesCustomerSubscribeToProduct | String token String customerNumber String productNumber String subscriptionTitle out MethodResult result |
Check if a customer is a subscriber. While the customer number is required the product number and subscription title can be left blank. If left blank the method checks if the customer is a subscriber, in general, regardless of product or subscription category, respectively. This method returns a boolean value. |
GetSubscription | String token String subscriptionTitle out MethodResult result |
Get a subscription object by title. If a subscripton does not contain a value for a certain dimension an empty dimension (one with all values zeros and empty strings) is returned. This method returns an object of type Subscription. |
GetSubscriptionEntry | String token int subscriptionEntryID out MethodResult result |
Get a subscription entry by its id. This method returns an object of type SubscriptionEntry. |
GetSubscriptions | String token out MethodResult result |
Get all subscription. If a subscription does not contain a value for a certain dimension an empty dimension (one with all values zeros and empty strings) is returned. This method returns an array of objects of type Subscription. |
SaveSubscription | String token Subscription subscription |
This method inserts a subscription if it does not already exist. If subscription already exists it is updated if needed. The CreateSubscriptionEntry(…) method calls this method internally. This method returns an object of type MethodResult. |
SaveSubscriptionEntry | String token SubscriptionEntry subscriptionEntry |
This method inserts a subscription entry if it does not already exist (based on id). If subscription already exists it is updated if needed. This method returns an object of type MethodResult. |
ValidateSubscription | String token Subscription subscription |
The SaveSubscription(…) method calls this method internally. This method returns an object of type MethodResult. |
ValidateSubscriptionEntry | String token SubscriptionEntry subscriptionEntry |
The SaveSubscriptionEntry(…) method calls this method internally. This method returns an object of type MethodResult. |
Create Subscription Entry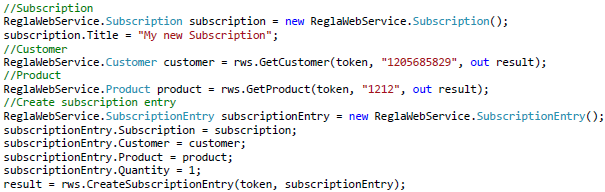
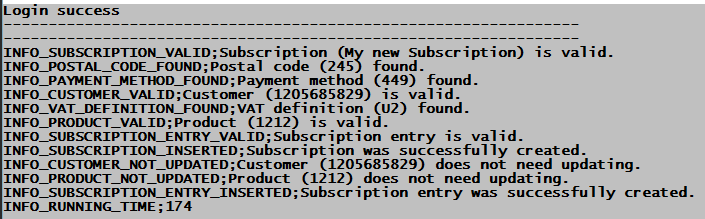
Project
Method | Parameters | Description |
GetProject | String token int projectId Boolean includeProjectAccountingEntries out MethodResult result |
Get project by id. Parameter includeProjectAccountingEntries determines whether to get entries associated with project. This method returns an object of type Project. |
GetProjects | String token Boolean includeProjectAccountingEntries out MethodResult result |
Get all projects. Parameter includeProjectAccountingEntries determines whether to get entries associated with project. This method returns an array of objects of type Project. |
GetProjectsByStatuses | String token ProjectStatus[] statuses, Boolean includeProjectAccountingEntries out MethodResult result |
Get all projects having status in statuses. Parameter includeProjectAccountingEntries determines whether to get entries associated with project. This method returns an array of objects of type Project. |
SaveProject | String token Project project |
This method inserts a project if it does not already exist. If project already exists it is updated if needed. This method returns an object of type MethodResult. |
ValidateProject | String token Project project |
Validate a project. The SaveProject method calls this method internally. This method returns an object of type MethodResult. |
ProjectAccountingEntry
Method | Parameters | Description |
GetProjectAccountingEntries | String token DateTime from DateTime to String employeeUserName out MethodResult result |
Gets project accounting entries by employee and period. An empty string for employee user name returns entries for all employees. This method returns an array of objects of type ProjectAccountingEntry. |
GetProjectAccountingEntriesByStatus | String token DateTime from DateTime to String employeeUserName ProjectAccountingEntryStatus status out MethodResult result |
Gets project accounting entries by employee, period and status. An empty string for employee user name returns entries for all employees. This method returns an array of objects of type ProjectAccountingEntry. |
GetProjectAccountingEntry | String token int id out MethodResult result |
Get a project accounting entry by id. This method returns an object of type ProjectAccountingEntry. |
SaveProjectAccountingEntry | String token ProjectAccountingEntry projectAccountingEntry |
This method inserts a project accounting entry if it does not already exist. If project accounting entry already exists it is updated if needed. This method returns an object of type MethodResult. |
SearchProjectAccountingEntries | String token String search int indexFrom int maxRecordCount out MethodResult result |
Searches project accounting entries for a company. Multiple search values can be separated by ",", ";" or "+". An empty search string returns all project accounting entries. The search string can contain parts of project name, project description, project customer number or customer name and project accounting entry description or notes. indexFrom is a zero based start index. maxRecordCount defines how many records to return max. The result array contains the current page number (INFO_SEARCH_PAGE_NUMBER), total page count (INFO_SEARCH_PAGE_COUNT), the total number of project accounting entries (INFO_SEARCH_TOTAL_COUNT) and number of project accounting entries returned by this call to the method (INFO_SEARCH_RECORD_COUNT), respectively. This method returns an array of objects of type ProjectAccountingEntry. |
SearchProjectAccountingEntriesModified | String token String search DateTime modifie int indexFrom int maxRecordCount out MethodResult result |
This method adds a from time to method SearchProjectAccountingEntries (…) and only returns project accounting entries that have changed since that time. This method returns an array of objects of type ProjectAccountingEntry. |
ValidateProjectAccountingEntry | String token ProjectAccountingEntry projectAccountingEntry |
Validate a project accounting entry. The SaveProjectAccountingEntry(…) method calls this method internally. This method returns an object of type MethodResult. |
ProjectAccountingProductEntry
Method | Parameters | Description |
GetProjectAccountingProductEntries | String token DateTime from DateTime to String employeeUserName out MethodResult result |
Gets project accounting product entries by employee and period. An empty string for employee user name returns entries for all employees. This method returns an array of objects of type ProjectAccountingProductEntry. |
GetProjectAccountingProductEntriesByProjectID | String token int projectID out MethodResult result |
Gets project accounting product entries by project id. This method returns an array of objects of type ProjectAccountingProductEntry. |
GetProjectAccountingProductEntry | String token int projectAccountingProductEntryId out MethodResult result |
Get a project accounting entry by id. This method returns an object of type ProjectAccountingProductEntry. |
SaveProjectAccountingProductEntry | String token ProjectAccountingProductEntry projectAccountingProductEntry |
This method inserts a project accounting product entry if it does not already exist. If project accounting product entry already exists it is updated if needed. This method returns an object of type MethodResult. |
SearchProjectAccountingProductEntries | String token String search int indexFrom int maxRecordCount out MethodResult result |
Searches project accounting product entries for a company. Multiple search values can be separated by ",", ";" or "+". An empty search string returns all project accounting product entries. The search string can contain parts of project name, project description, project customer number or customer name and project accounting product entry product number or product name and description . indexFrom is a zero based start index. maxRecordCount defines how many records to return max. The result array contains the current page number (INFO_SEARCH_PAGE_NUMBER), total page count (INFO_SEARCH_PAGE_COUNT), the total number of project accounting product entries (INFO_SEARCH_TOTAL_COUNT) and number of project accounting product entries returned by this call to the method (INFO_SEARCH_RECORD_COUNT), respectively. This method returns an array of objects of type ProjectAccountingProductEntry. |
SearchProjectAccountingProductEntriesModified | String token String search DateTime modified int indexFrom int maxRecordCount out MethodResult result |
This method adds a from time to method SearchProjectAccountingProductEntries (…) and only returns project accounting product entries that have changed since that time. This method returns an array of objects of type ProjectAccountingProductEntry. |
ValidateProjectAccountingProductEntry | String token ProjectAccountingProductEntry projectAccountingProductEntry |
Validate a project accounting product entry. The SaveProjectAccountingProductEntry(…) method calls this method internally. This method returns an object of type MethodResult. |
Accounting
Method | Parameters | Description |
GetAccountingKey | String token TransactionCategory category int serial int year out MethodResult result | Get accounting key by its key. This method returns an object of type AccountingKey. |
GetAccountingKeys | String token out MethodResult result | Get all active accounting keys. This method returns an array of objects of type AccountingKey. |
GetNextTransaction | String token TransactionCategory category DateTime date out MethodResult result |
Get next accounting transaction. Use this method to create a new transaction. This method returns an object of type Transaction. |
GetTransaction | String token TransactionCategory category int serial int year out MethodResult result |
Get an accounting transaction by category, serial and year. This method returns an object of type Transaction. |
GetTransactionCategories | String token out MethodResult result |
Get all transaction categories. This method returns an array of objects of type TransactionCategory. |
GetTransactionCategory | String token String key out MethodResult result |
Get transaction category by its key. This method returns an object of type TransactionCategory. |
GetTransactions | String token DateTime from DateTime to int category int serialFrom int serialTo Decimal amount Boolean includeTransactionEntries out MethodResult result |
Get transactions by various criteria. This method returns an array of object of type Transaction. |
SaveTransaction | String token WebServices.DTO.Transaction transaction |
This method inserts an accounting transaction if it does not already exist. If accounting transaction already exists it is updated if needed. This method returns an object of type MethodResult. |
ValidateTransaction | String token WebServices.DTO.Transaction transaction |
Validate a transaction. The SaveTransaction method calls this method internally. This method returns an object of type MethodResult. |
StockRoom
Method | Parameters | Description |
GetProductStockQuantity | String token String productNumber int stockRoomID out MethodResult result |
Get product stock quantity for a particular stock room. A stock room id of zero returns stock quantity regardless of stock room. This method returns a value of type double. |
GetStockRoom | String token int stockRoomID out MethodResult result |
Get stock room by its id. This method returns an object of type StockRoom. |
GetStockRoomByDepartment | String token int stockRoomID out MethodResult result |
Get stock room by department id. This method returns an object of type StockRoom. |
GetStockRooms | String token out MethodResult result |
Get all stock rooms. This method returns an array of objects of type StockRoom. |
StockCount
Method | Parameters | Description |
GetStockCountByDay | String token DateTime day out MethodResult result |
Get stock count by day. This method returns an array of objects of type StockCount. |
GetStockCountByID | String token int stockCountID out MethodResult result |
Get a stock count object by its id. This method returns an object of type StockCount. |
<="" td=""> | String token DateTime day String productNumber out MethodResult result |
Get stock count by product number. This method returns an array of objects of type StockCount. |
GetStockCountByStockRoom | String token DateTime day int stockRoomID out MethodResult result |
Get all stock count by StockRoom. This method returns an array of objects of type StockCount. |
SaveStockCount | String token StockCount stockCount bool add |
This method inserts a stock count if it does not already exist. If stock count already exists, based on its id, it is updated if needed. If the value of parameter add is true, quantity is added to existing quantity, otherwise the value of quantity is simply updated. This method returns an object of type MethodResult. |
ProductBarcode
Method | Parameters | Description |
GetAllProductBarcodes | String token out MethodResult result |
Get barcodes for all products. This method returns an array of objects of type ProductBarcode. |
GetProductBarcodesByProductID | String token int productID out MethodResult result |
Get product barcodes by product id. This method returns an array of objects of type ProductBarcode. |
GetProductBarcodesByProductNumber | String token String productNumber out MethodResult result |
Get product barcodes by product number. This method returns an array of objects of type ProductBarcode. |